티스토리 뷰
지날 글 (2023.02.12 - [Remote Sensing] - AI 모델을 사용한 도시 홍수 취약성 지도 제작(1) 에서 도시 홍수와 도시 홍수를 매핑하기 위한 데이터 기반 모델의 적용을 소개했습니다 . 이 글은 Geomatics, Natural Hazards and Risk에 게시된 " Towards urban flood susceptibility mapping using data-driven models in Berlin, Germany " 논문을 파이썬 코드로 요약하고 설명합니다 .
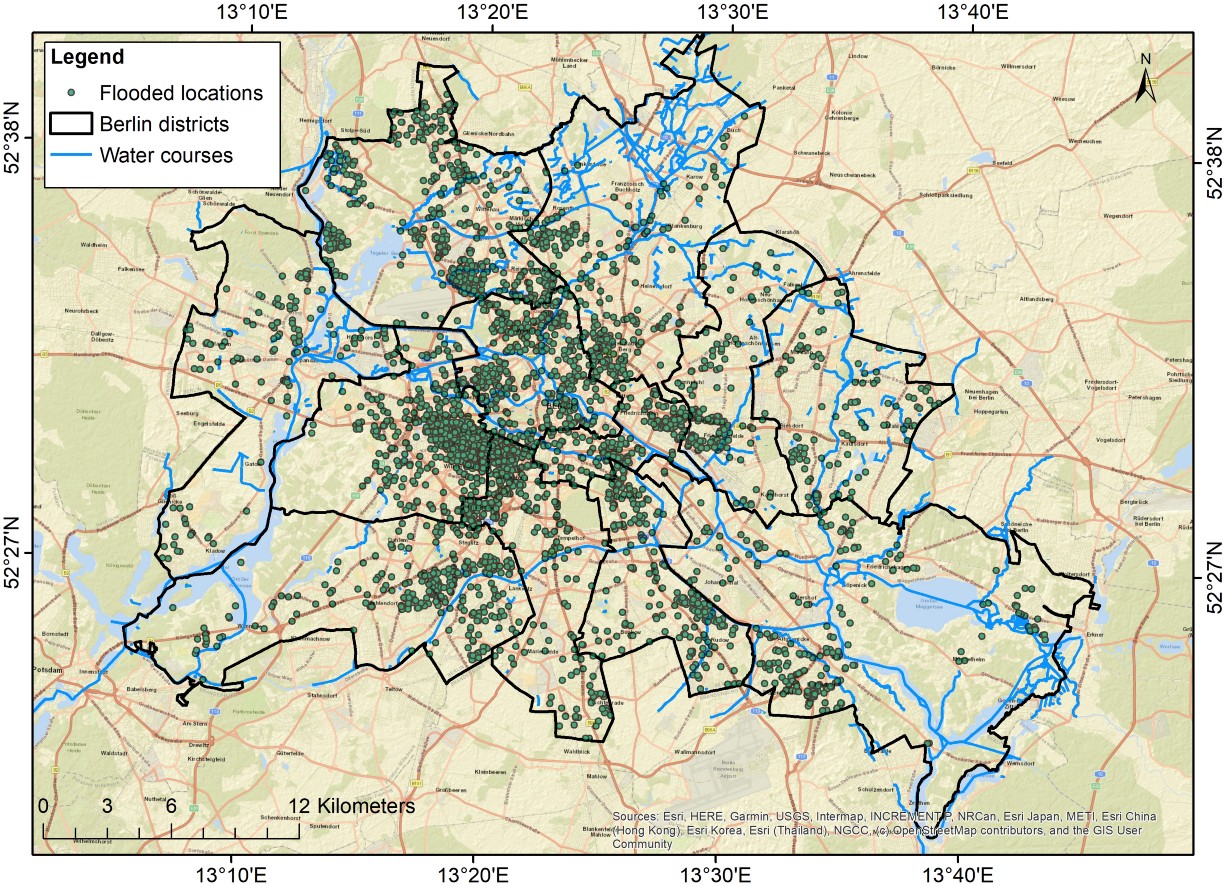
도시 하천 범람은 저지대나 유거수가 쌓이는 경향이 있는 지형적 함몰부에서 발생할 것으로 예상됩니다.
따라서 고도, 경사, 곡률 및 지형적 습윤 지수(TWI)와 같은 지형적 특징은 잠재적으로 홍수 위험이 증가했음을 나타냅니다. 또한, 우수 배수 시스템 용량 초과로 인해 도시 하천 범람이 발생합니다. 과도한 유거수는 도로망을 통해 이동하여 이를 우선 경로로 전환합니다.
따라서 위치와 가장 가까운 도로, 협곡 및 수로 사이의 거리는 홍수 취약성을 매핑하는 데 중요한 것으로 간주됩니다. 마지막으로 도시 홍수는 집중호우로 인해 발생한다.
따라서 강우량도 예측 특성으로 포함되어야 합니다. 우리는 강우량을 나타내기 위해 일일 최대 강우 깊이와 극한 강우 사건의 빈도를 사용했습니다. 그림 2는 고려된 예측 기능을 보여줍니다. 이러한 예측 기능은 Arcmap, QGIS 또는 Python에서 계산할 수 있습니다. 본 논문은 Convolutioanl Neural Network(CNN)(raster-based model)과 Random Forest(RF), Support Vector Machine(SVM), 인공신경망(ANN)(point-based models)과 같은 전통적인 기계 학습 알고리즘을 비교하였다.
홍수 취약성 예측지도를 제작에서 세웠던 가설은 CNN이 다른 모델보다 우수하다는 것입니다.
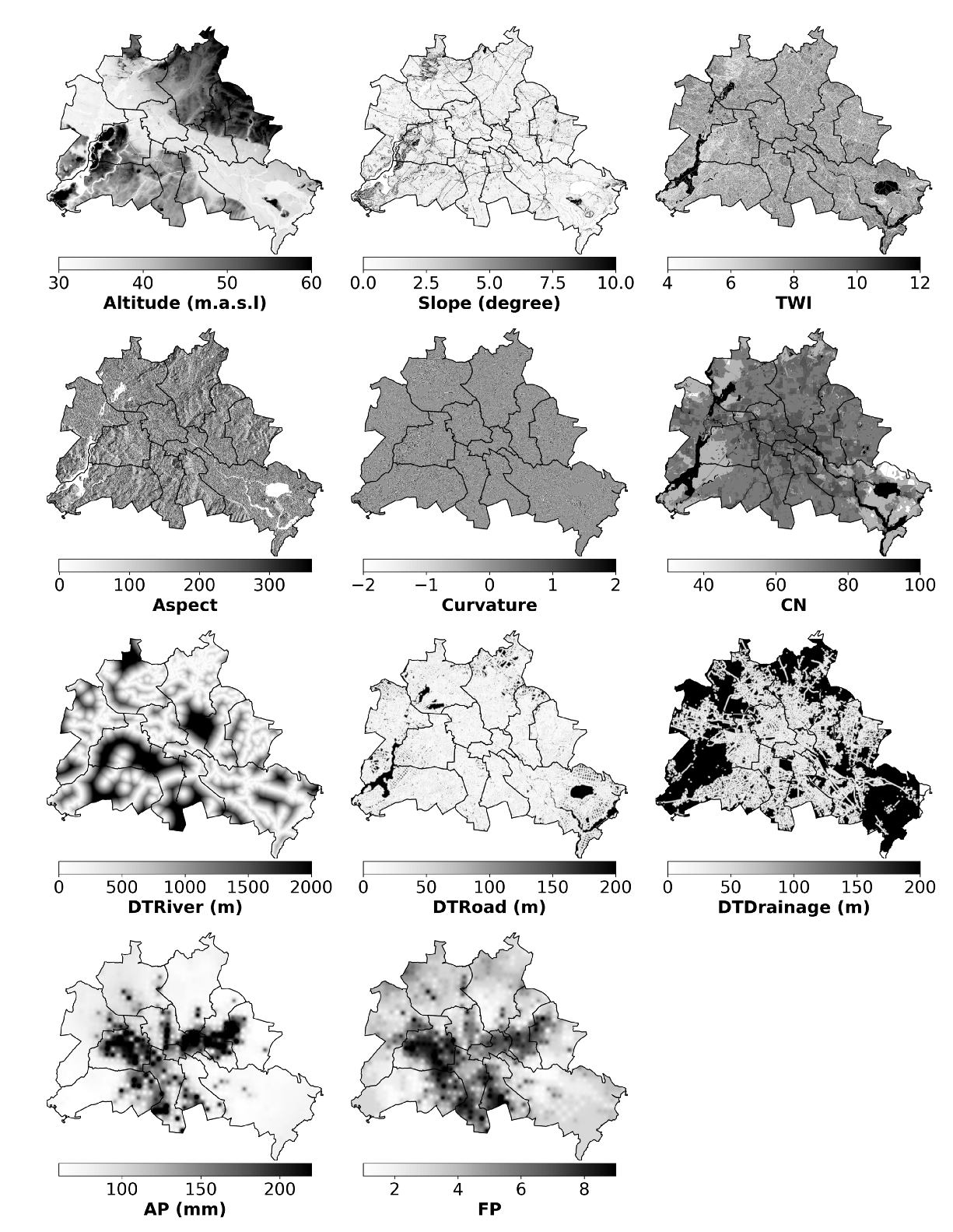
이 문서에서는 포인트 기반 모델에 대한 데이터를 준비하는 방법을 설명합니다. geopandas를 사용하여 python에서 홍수 및 비침수 지점을 포함하는 shapefile을 읽을 수 있습니다. 그런 다음 각 예측 기능에 대해 빈 열을 추가해야 합니다.
<코드 1>
# Import used packages
import geopandas as gpd # used to read the shapfile
import rasterio as rio # used to read the raster (.tif) files
from rasterio.plot import show # used to make plots using rasterio
import matplotlib.pyplot as plt #to make plots using matplotlib
# Read your point shapefiles (Flooded and Non Flooded locations)
points=gpd.read_file('Points.shp')
# make columns to extract the values of each predictive feature
# for each point.
points['DEM']=0 #
points['Slope']=0
points['Aspect']=0
points['Curvature']=0
points['TWI']=0
points['DTDrainage']=0
points['DTRoad']=0
points['DTRiver']=0
points['CN']=0
points['AP']=0 #Max daily precipitation
points['FP']=0 #Frequency of extreme precipitation event.
그런 다음 rasterio 또는 gdal을 사용하여 예측 기능(래스터 이미지)을 열고 NumPy 배열로 읽어야 Python에서 작업할 수 있습니다.
<코드 2>
#The predictive features are in raster format so we use rasterio package to
#read them and convert them to numpy array
DEM_raster=rio.open('DEM.tif')
DEM_arr=DEM_raster.read(1)
Slope_raster=rio.open('Slope.tif')
Slope_arr=Slope_raster.read(1)
Aspect_raster=rio.open('Aspect.tif')
Aspect_arr=Aspect_raster.read(1)
Curvature_raster=rio.open('Curvature.tif')
Curvature_arr=Curvature_raster.read(1)
TWI_raster=rio.open('TWI.tif')
TWI_arr=TWI_raster.read(1)
DTRoad_raster=rio.open('DTRoad.tif')
DTRoad_arr=DTRoad_raster.read(1)
DTRiver_raster=rio.open('DTRiver.tif')
DTRiver_arr=DTRiver_raster.read(1)
DTDrainage_raster=rio.open('DTDrainage.tif')
DTDrainage_arr=DTDrainage_raster.read(1)
CN_raster=rio.open('CN.tif')
CN_arr=CN_raster.read(1)
AP_raster=rio.open('AP.tif')
AP_arr=AP_raster.read(1)
FP_raster=rio.open('FP.tif')
FP_arr=FP_raster.read(1)
#show point and raster on a matplotlib plot
fig, ax = plt.subplots(figsize=(12,12))
points.plot(ax=ax, color='orangered')
show(DEM_raster, ax=ax)
이제 우리는 geopandas를 사용하여 포인트를 데이터 프레임으로 읽고 rasterio를 사용하여 예측 기능을 열고 숫자 배열로 읽었습니다. 다음으로 침수된 지점과 비침수된 지점에 대한 예측 특성 값을 추출해야 합니다.
<코드 3>
# Extracting the raster values to the points shapefile
# count=0
for index,row in points.iterrows(): #iterate over the points in the shapefile
longitude=row['geometry'].x #get the longitude of the point
latitude=row['geometry'].y #get the latitude of the point
#print("Longitude="+str(longitude))
#print(count)
#count +=1
rowIndex, colIndex=DEM_raster.index(longitude,latitude) # the corresponding pixel to the point (longitude,latitude)
# Extract the raster values at the point location
points['DEM'].loc[index]=DEM_arr[rowIndex, colIndex]
points['Slope'].loc[index]=Slope_arr[rowIndex, colIndex]
points['Aspect'].loc[index]=Aspect_arr[rowIndex, colIndex]
points['Curvature'].loc[index]=Curvature_arr[rowIndex, colIndex]
points['DTRoad'].loc[index]=DTRoad_arr[rowIndex, colIndex]
points['DTRiver'].loc[index]=DTRiver_arr[rowIndex, colIndex]
points['DTDrainage'].loc[index]=DTDrainage_arr[rowIndex, colIndex]
points['TWI'].loc[index]=TWI_arr[rowIndex, colIndex]
points['CN'].loc[index]=CN_arr[rowIndex, colIndex]
points['AP'].loc[index]=AP_arr[rowIndex, colIndex]
points['FP'].loc[index]=FP_arr[rowIndex, colIndex]
points.head() # to have a look on the calculated fields.
# Save the points file
points.to_file('points_data.shp') # save as a shapfile
# or
points.to_pickle('points_data.pkl') # save as a pickle.
이제 침수 위치와 비침수 위치를 지정하고 각 포인트의 예측 기능 값을 포함하는 포인트 shapefile이 있습니다. 다음 기사에서는 도시 홍수 감수성을 매핑하기 위해 포인트 기반 모델을 구현하는 방법에 대해 설명합니다.
2023.02.18 - [Remote Sensing] - AI 모델을 사용한 도시 홍수 취약성 지도 제작(3)
'Remote Sensing' 카테고리의 다른 글
AI 모델을 사용한 도시 홍수 취약성 지도 제작(3) (0) | 2023.02.18 |
---|---|
위성사진과 python을 이용하여 토지이용 변화를 정량화 하기 (0) | 2023.02.17 |
AI 모델을 사용한 도시 홍수 취약성 지도 제작(1) (0) | 2023.02.14 |
Rasterio 파이썬 패키지를 사용하여 공간 래스터 데이터 처리 (0) | 2023.02.13 |
우리나라 Landsat 위성 이동경로 - WRS(Worldwide Reference System) 2 (0) | 2022.09.09 |